Docker has changed dramatically the way we develop applications. Thanks to it, it is really easy for everyone to run a complex application with a single command, without having to worry about the inner details like dependencies. These advantages are even greater when working on a team or enterprise context. I still remember being like the first 3 days when I joined my current company, configuring the project and all the related libraries and tools. Docker make it such much easier, faster and consistent.
But everything comes with a price. There is an extra complexity of maintaining all the Docker stuff. Also some things that were very easy in a normal development environment running locally, like debugging your application from your IDE, now requires some extra configuration. And in case of getting Xdebug to work, its not an easy task. I couldn't find a single guide that have all the steps from start to finish. Thats why I decided to write this article. It will guide you to step by step through the process of installing and configuring Xdebug and PHPStorm with a Dockerized Symfony 4 application.
Pre-requisites
Instruct XDebug to connect to host.docker.internal for command line execution or whenever “connect back” is not possible. Set PHPIDECONFIG env variable to serverName=localhost. This will tell your PhpStorm which server configuration to use. See next step for details. FROM php: fpm RUN docker-php-ext-install pdo pdomysql RUN pecl install xdebug && docker-php-ext-enable xdebug xdebug is installed through pecl, which is provided as part of the official PHP image.
- This was tested on an Ubuntu 18.04 machine with PHPStorm 2018.1.4 and latest versions of Docker and Docker Compose. Some things might work a little different in other Operating Systems.
- I assume you have a basic Knowledge of Docker, PHP and XDebug.
- You can clone this repository as base to follow this gude as it contains a basic Symfony Flex application with all the Docker stuff explained in this article included.
Step 1 - Dockerize the application
Of course, to be able to use Xdebug you must install it on your Docker container.The way to do this, will depend of your base image. I always use alpine based images. I wont enter in detail about how to Dockerize a Symfony application. You can follow along with the Dockerfile included in the demo repository.
Here is the relevant excerpt of the Dockerfile that installs Xdebug:
I dont want have to have a separate Dockerfile for development and production, so I have defined a build argument that will tell whether we want to install Xdebug or not.
Then, on my Docker-compose file I have the following definition for my application:
See for the full docker-compose file.
Nothing really fancy about this. The important bit is the 'env_file' instruction which tells Compose to load environment variables from a '.env' file, which is the standard way for Symfony 4 applications.
We will use that file to add some required environment variables for Xdebug. If you prefer in you can also add directly to the docker-compose file using the 'environment' section.
Environment Variables
We will define the following environment variables:
- PHP_IDE_CONFIG - This variable defines the server configuration associated with the application. More on this later.
- XDEBUG_CONFIG - This variable allows to define some Xdebug configurations. The 'remote host' is the private ip of your host machine (the one your PHPStorm is running). The 'remote_port' is the port that PHPStorm will be listening for incoming Xdebug connections. These two settings allow PHPStorm and Xdebug to communicate. It wont work without this.
We will add them to our '.env' file like this:
And thats it in terms of code.
Next lets dig into PHPStorm configurations.
PHPStorm configurations
The first thing you should do is to check your Debug settings. In PHPStorm, go to File -> Settings -> Languages and Frameworks -> PHP > Debug.
Make sure you have the some port that you have configured previously in 'XDEBUG_CONFIG' environment variable:
Next, we need to configure a server. This is how PHPStorm will map the file paths in your local system to the ones in your container.
Go to File -> Settings -> Languages and Frameworks -> PHP -> Servers
Give a name to your server. It should match the value you have defined in your 'PHP_IDE_CONFIG' environment variable. We will call it 'symfony-demo'.
The 'host' and 'port' is how will access your application. In my case is localhost:8888.
And then the 'Path mappings'.
In the 'Project files' section you have to map the root path of your application to the path inside the container. In my case its '/var/www/app'.
Click 'Apply' to save your configurations.
The last part is to configure the remote debugger of your project.
On the top right, click on 'edit configurations':
Docker Php Fpm Xdebug
Click in the green 'plus' sign at the top left and select 'PHP Remote Debug' from the list.
Now configure it like this:
Make sure you associate it with the previously created 'server' definition. Use 'PHPSTORM' as idekey.
Your IDE should be now correctly configured. Lets test.
Testing
Open 'src/Controllers/HelloController.php' and place a breakpoint in the 'hello' method.
Start your Docker container with
docker-compose up
Then click on 'Start Listening for PHP Debug connections' icon on top right corner of PHPStorm.
- Open http://localhost:8888?XDEBUG_SESSION_START=PHPSTORM
If everything went well you should see the execution stop at your breakpoint.
And thats it. You should now have a fully configured development environment with Docker and Xdebug integrated with PHPStorm IDE.
If you have any issues or questions feel free to comment bellow or in the GitHub Repository.
Thank you and good debugging ;)
FeaturesNewsletterTutorialsSo, you’ve decided to try something new today and started a project from scratch. Your first step will be to set up a development environment: at the bare minimum, you’d want to run a web server and a PHP interpreter (preferably – with the debugging engine installed).
With Docker, you can start developing, running, and debugging your code in a matter of minutes!
Probably the easiest way to integrate Docker with PhpStorm is to use the PhpStorm Docker registry. It provides a selection of preconfigured Docker images curated by the PhpStorm team, which cover the most common PHP development needs.
Docker Php Xdebug 3
Before you proceed, make sure that you have Docker installed on your machine: see how to do it on Windows and on macOS.
Defining the environment
To get started, we create a new project in PhpStorm. Next, we create a new file named docker-compose.yml , which will describe the configuration of the services comprising our app. In our case, it will be a single webserver service:
As you can see, we use the preconfigured Docker image comprising the Apache web server and PHP 7.1 with Xdebug.
Note that we use the host.docker.internal value to refer to the remote host. In Docker for Windows and Docker for Mac, it automatically resolves to the internal address of the host, letting you easily connect to it from the container.
An important note for Linux users: host.docker.internal on Linux is currently not supported. You’ll have to use your local machine’s hostname instead (to find out what your machine’s hostname is, simply execute hostname in Terminal).
The corresponding environment configuration section for Linux will read as follows:
See here for more details and possible workarounds.
Our environment is fully described:
Debug Php Vscode Remote
We can now start using it by creating a dedicated run/debug configuration.

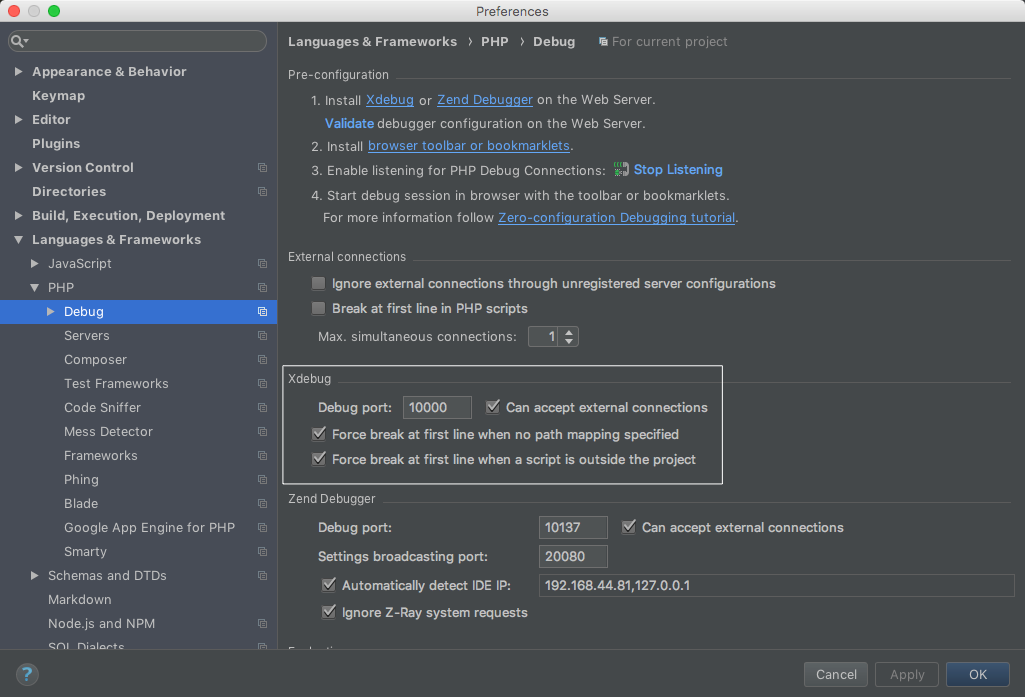
Creating a run/debug configuration
Right-click docker-compose.yml and select Create… from the context menu:
In the dialog that opens, provide the name of the configuration and apply your changes:
You can now start the configuration from the toolbar:
PhpStorm will automatically download the required image and start the web server:
That’s it: we’ve got everything ready for running and debugging our code!
Running and debugging code
Let’s ensure that everything works as expected. To do this, we’ll create the most simple Hello world PHP file and try to debug it following the PhpStorm Zero-Configuration Debugging approach.
Since we already have Xdebug installed and configured, this will only require that you:
- Have a debugging extension installed and enabled for your browser:
- Set a breakpoint in your code:
- Enable listening to incoming debug connections in PhpStorm:
Now, simply open the page in the browser, and the debugging session will be started automatically:
We encourage you to further explore the PhpStorm Docker registry: while we’ve looked at a very simple case, you can use the described technique to provide your environment with, for example, a database, or an sftp server.
Using these Docker images will save you a lot of effort and let you start coding in a matter of a minute, or even less!
If you’d like to learn more about Docker and how to use it in PhpStorm, make sure to check out the excellent tutorial series by Pascal Landau, and PhpStorm documentation, of course.
Your JetBrains PhpStorm Team
The Drive to Develop
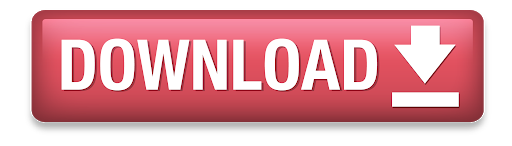